Resources in your Azure subscriptions can be created or modified in different ways. In one of my last projects I had the task to implement an easy on demand executable script which performs some actions in a resource group based on a parameter.
One way to access resources is with Azure powershell commands. And because the parameter data should be handed over through a web hook from Azure DevOps, the proposed solution was an Azure function with Powershell runtime. The steps to do are:
- Create a function app and a function
- Activate the managed identity
- Set RBAC for the identity
- Modify the function for Azure Powershell
So there are some things to do, let’s dive in…
Create the function
For the sake of simplicity, I created the function over the portal and not project based within Visual Studio.
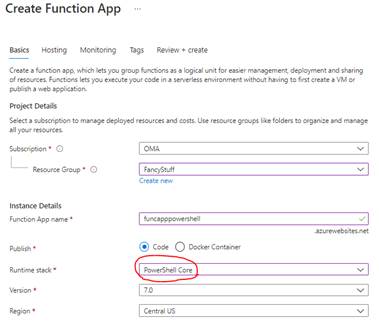
The remaining options to create the function app can be set with default options
Next, add the function with triggered by http call:
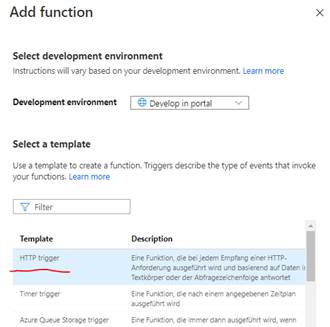
Activate the managed identity
A fantastic way to manage the access to resources is to work with system or user assigned managed identities. This is a feature available for some resources like an App Service or a Function app, represented internally through the known service principals. But in this case, we don’t have to take care about the creation, it’s just a click in the identity settings of your function app:
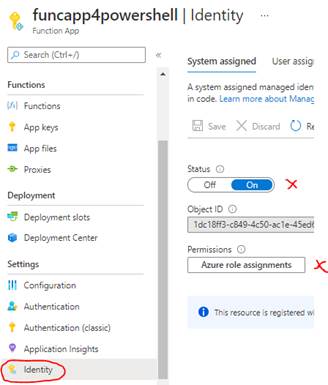
Selecting the tab for System assigned, switching it on and saving activates the identity. A user assigned identity is a standalone resource and could be shared with other resources.
Set RBAC for the identity
In the same area we can navigate to the roles assignments to add the needed permissions.
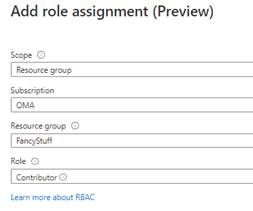
After choosing scope and Role, the function app can act over the system identity and modify (or maybe just read) the Azure resources.
Modify the function for Azure Powershell
The last step is to add the Azure PowerShell modules for the function app.
Under Development Tools -> Advanced Tools you can access the data from the app:
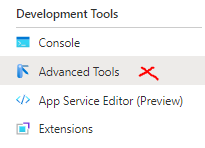
With a Debug console (Powershell) we switch the folder site\wwwroot and modify the file requirements.psd1
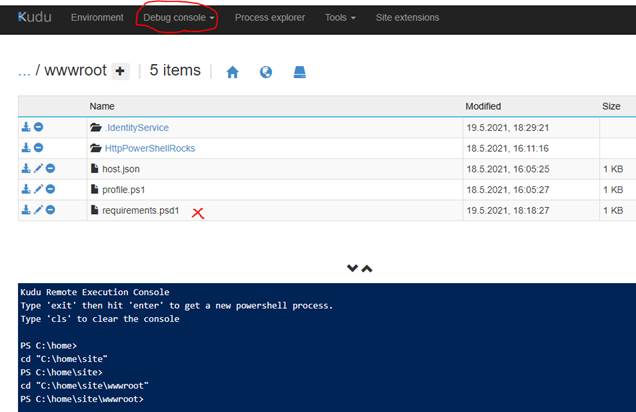
Normally it’s just removing the comment hashtag:
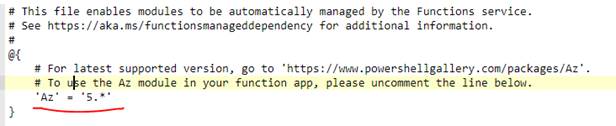
In combination with the enabled dependeny management in the file host.json, the required modules can be retrieved from the PowerShell gallery:
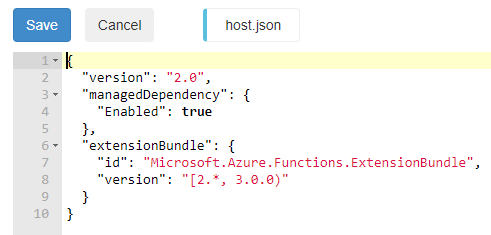
After a restart of the function app it “understands” Azure Powershell scripts.
Code used for the example, simply adding a tag:
using namespace System.Net
# Input bindings are passed in via param block.
param($Request, $TriggerMetadata)
# Interact with query parameters or the body of the request.
$name = $Request.Query.Name
if (-not $name) {
$name = $Request.Body.Name
}
Set-AzResourceGroup -Name $name -Tag @{Department="IT"}
$body = Get-AzResourceGroup -Name $name
# Associate values to output bindings by calling 'Push-OutputBinding'.
Push-OutputBinding -Name Response -Value ([HttpResponseContext]@{
StatusCode = [HttpStatusCode]::OK
Body = $body
})
Test it with a body or query string parameter directly in the portal:
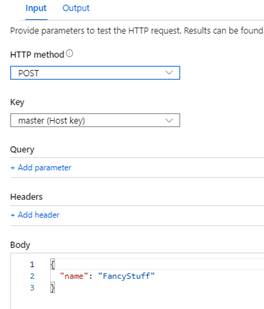
Use cases
It’s serverless, and it can simply do some work for you, on demand or maybe timer triggered. So the use cases are rich. It could be a regulary working clean up script without the “overhead” of an Automation Account or maybe a task triggered through an Action Group from an Alert, just to have some examples.
Have fun with functions (-: